FAQ PHP
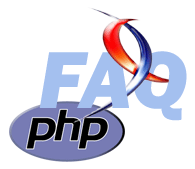
FAQ PHPConsultez toutes les FAQ
Nombre d'auteurs : 68, nombre de questions : 580, dernière mise à jour : 18 septembre 2021

Les documents OpenOffice sont des archives ZIP de documents XML. À ce titre, il faut avoir activé les extensions ZIP et DOM pour pouvoir lire ces documents en PHP 5.
Lien : Comment installer une extension pour PHP ?
Lien : Qu'est-ce que l'extension ZIP ?
OpenOffice utilise des fichiers ZIP contenant des fichiers XML.
- /META-INF/manifest.xml : Décrit le type du document principal (Text, Calc, Presentation, etc.) ainsi que de tous les autres éléments de l'archive ;
- /content.xml : Le document principal, il peut contenir du texte, un classeur Spreadsheet, des slides Presentation, etc.
<?xml version="1.0" encoding="utf-8" ?>
<
manifest
:
manifest
xmlns
:
manifest
=
"urn:oasis:names:tc:opendocument:xmlns:manifest:1.0"
>
<
manifest
:
file-entry
manifest
:
media-type
=
"application/vnd.oasis.opendocument.text"
manifest
:
full-path
=
"/"
/>
<
manifest
:
file-entry
manifest
:
media-type
=
"text/xml"
manifest
:
full-path
=
"content.xml"
/>
</
manifest
:
manifest>
<?xml version="1.0" encoding="utf-8" ?>
<
office
:
document-content
xmlns
:
office
=
"urn:oasis:names:tc:opendocument:xmlns:office:1.0"
xmlns
:
text
=
"urn:oasis:names:tc:opendocument:xmlns:text:1.0"
>
<
office
:
body>
<
office
:
text>
<
text
:
p>
Hello world!</
text
:
p>
</
office
:
text>
</
office
:
body>
</
office
:
document-content>
Voici le code nécessaire pour créer de toutes pièces un document OpenOffice Text en PHP 5 :
<?php
$manifest
=
new
DOMDocument('1.0'
,
'utf-8'
);
$XMLManifest
=
$manifest
->
createElement('manifest:manifest'
);
$XMLManifest
->
setAttribute('xmlns:manifest'
,
'urn:oasis:names:tc:opendocument:xmlns:manifest:1.0'
);
$manifest
->
appendChild($XMLManifest
);
$XMLFileEntry
=
$manifest
->
createElement('manifest:file-entry'
);
$XMLFileEntry
->
setAttribute('manifest:media-type'
,
'application/vnd.oasis.opendocument.text'
);
$XMLFileEntry
->
setAttribute('manifest:full-path'
,
'/'
);
$XMLManifest
->
appendChild($XMLFileEntry
);
$XMLFileEntry
=
$manifest
->
createElement('manifest:file-entry'
);
$XMLFileEntry
->
setAttribute('manifest:media-type'
,
'text/xml'
);
$XMLFileEntry
->
setAttribute('manifest:full-path'
,
'content.xml'
);
$XMLManifest
->
appendChild($XMLFileEntry
);
$content
=
new
DOMDocument('1.0'
,
'utf-8'
);
$XMLOfficeContent
=
$content
->
createElement('office:document-content'
);
$XMLOfficeContent
->
setAttribute('xmlns:office'
,
'urn:oasis:names:tc:opendocument:xmlns:office:1.0'
);
$XMLOfficeContent
->
setAttribute('xmlns:text'
,
'urn:oasis:names:tc:opendocument:xmlns:text:1.0'
);
$content
->
appendChild($XMLOfficeContent
);
$XMLOfficeBody
=
$content
->
createElement('office:body'
);
$XMLOfficeContent
->
appendChild($XMLOfficeBody
);
$XMLOfficeText
=
$content
->
createElement('office:text'
);
$XMLOfficeBody
->
appendChild($XMLOfficeText
);
$XMLText
=
$content
->
createElement('text:p'
,
utf8_encode("Hello world!"
));
$XMLOfficeText
->
appendChild($XMLText
);
$document
=
new
ZipArchive();
$document
->
open('hello-world.odt'
,
ZIPARCHIVE::
OVERWRITE);
$document
->
addFromString('META-INF/manifest.xml'
,
$manifest
->
saveXML());
$document
->
addFromString('content.xml'
,
$content
->
saveXML());
$document
->
close();
?>
Lien : Que faut-il pour lire des documents OpenDocument en PHP ?
Un paragraphe de texte est un élément "p" contenant directement le texte (pas de run puis text comme dans OpenXML). Cet élément est contenu dans la racine "text" du document (dans le cas d'un document Text).
$XMLText
=
$content
->
createElement('
text:p
'
,
utf8_encode("
Hello world!
"
));
$XMLOfficeText
->
appendChild($XMLText
);
<?php
$content
=
new
DOMDocument('1.0'
,
'utf-8'
);
$XMLDocumentContent
=
$content
->
createElement('office:document-content'
);
$XMLDocumentContent
->
setAttribute('xmlns:office'
,
'urn:oasis:names:tc:opendocument:xmlns:office:1.0'
);
$XMLDocumentContent
->
setAttribute('xmlns:text'
,
'urn:oasis:names:tc:opendocument:xmlns:text:1.0'
);
$content
->
appendChild($XMLDocumentContent
);
$XMLBody
=
$content
->
createElement('office:body'
);
$XMLDocumentContent
->
appendChild($XMLBody
);
$XMLText
=
$content
->
createElement('office:text'
);
$XMLBody
->
appendChild($XMLText
);
$XMLParagraph
=
$content
->
createElement('text:p'
);
$XMLText
->
appendChild($XMLParagraph
);
$XMLText
=
$content
->
createTextNode(utf8_encode("Du texte simple."
));
$XMLParagraph
->
appendChild($XMLText
);
$content
->
save('content.xml'
);
?>
OpenDocument utilise un système très similaire à HTML pour mettre le texte en forme : des éléments span insérés directement dans le paragraphe permettent de modifier la mise en forme d'une portion de texte. Il faut appliquer un style à chacun de ces éléments span.
<
office
:
automatic-styles>
<
style
:
style
style
:
name
=
"b"
style
:
family
=
"text"
>
<
style
:
text-properties
fo
:
font-weight
=
"bold"
/>
</
style
:
style>
</
office
:
automatic-styles>
<
text
:
p
text
:
style-name
=
"Standard"
>
Un paragraphe
<
text
:
span
text
:
style-name
=
"b"
>
mis en forme</
text
:
span>
.
</
text
:
p>
$XMLAutomaticStyles
=
$content
->
createElement('
office:automatic-styles
'
);
$XMLDocumentContent
->
appendChild($XMLAutomaticStyles
);
$XMLStyle
=
$content
->
createElement('
style:style
'
);
$XMLStyle
->
setAttribute('
style:name
'
,
'
b
'
);
$XMLStyle
->
setAttribute('
style:family
'
,
'
text
'
);
$XMLAutomaticStyles
->
appendChild($XMLStyle
);
$XMLTextProperties
=
$content
->
createElement('
style:text-properties
'
);
$XMLTextProperties
->
setAttribute('
fo:font-weight
'
,
'
bold
'
);
$XMLStyle
->
appendChild($XMLTextProperties
);
// ...
$XMLParagraph
=
$content
->
createElement('
text:p
'
);
$XMLText
->
appendChild($XMLParagraph
);
$XMLText
=
$content
->
createTextNode(utf8_encode("
Du texte
"
));
$XMLParagraph
->
appendChild($XMLText
);
$XMLText
=
$content
->
createElement('
text:span
'
,
utf8_encode("
mis en forme
"
));
$XMLText
->
setAttribute('
text:style-name
'
,
'
tests
'
);
$XMLParagraph
->
appendChild($XMLText
);
$XMLText
=
$content
->
createTextNode(utf8_encode("
.
"
));
$XMLParagraph
->
appendChild($XMLText
);
L'élément "office:automatic-styles" doit être ajouté à l'élément "office:document-content".
L'élément "text:p" doit être ajouté à un élément "office:text".
<?php
$content
=
new
DOMDocument('1.0'
,
'utf-8'
);
$XMLDocumentContent
=
$content
->
createElement('office:document-content'
);
$XMLDocumentContent
->
setAttribute('xmlns:office'
,
'urn:oasis:names:tc:opendocument:xmlns:office:1.0'
);
$XMLDocumentContent
->
setAttribute('xmlns:text'
,
'urn:oasis:names:tc:opendocument:xmlns:text:1.0'
);
$XMLDocumentContent
->
setAttribute('xmlns:fo'
,
'urn:oasis:names:tc:opendocument:xmlns:xsl-fo-compatible:1.0'
);
$XMLDocumentContent
->
setAttribute('xmlns:style'
,
'urn:oasis:names:tc:opendocument:xmlns:style:1.0'
);
$content
->
appendChild($XMLDocumentContent
);
$XMLAutomaticStyles
=
$content
->
createElement('office:automatic-styles'
);
$XMLDocumentContent
->
appendChild($XMLAutomaticStyles
);
$XMLStyle
=
$content
->
createElement('style:style'
);
$XMLStyle
->
setAttribute('style:name'
,
'tests'
);
$XMLStyle
->
setAttribute('style:family'
,
'text'
);
$XMLAutomaticStyles
->
appendChild($XMLStyle
);
$XMLTextProperties
=
$content
->
createElement('style:text-properties'
);
$XMLTextProperties
->
setAttribute('fo:font-family'
,
'Courier new'
);
$XMLTextProperties
->
setAttribute('fo:font-weight'
,
'bold'
);
$XMLTextProperties
->
setAttribute('fo:font-style'
,
'italic'
);
$XMLTextProperties
->
setAttribute('fo:color'
,
'#FF0000'
);
$XMLTextProperties
->
setAttribute('fo:background-color'
,
'#00FFFF'
);
$XMLTextProperties
->
setAttribute('style:text-underline-type'
,
'single'
);
$XMLStyle
->
appendChild($XMLTextProperties
);
$XMLBody
=
$content
->
createElement('office:body'
);
$XMLDocumentContent
->
appendChild($XMLBody
);
$XMLText
=
$content
->
createElement('office:text'
);
$XMLBody
->
appendChild($XMLText
);
$XMLParagraph
=
$content
->
createElement('text:p'
);
$XMLText
->
appendChild($XMLParagraph
);
$XMLText
=
$content
->
createTextNode(utf8_encode("Du texte "
));
$XMLParagraph
->
appendChild($XMLText
);
$XMLText
=
$content
->
createElement('text:span'
,
utf8_encode("mis en forme"
));
$XMLText
->
setAttribute('text:style-name'
,
'tests'
);
$XMLParagraph
->
appendChild($XMLText
);
$XMLText
=
$content
->
createTextNode(utf8_encode("."
));
$XMLParagraph
->
appendChild($XMLText
);
$content
->
save('content.xml'
);
?>